In the case of convertible laptops the keyboard and touchpad needs to be disabled if the laptop screen is being used as a tablet and you dont want accidental keypresses. This happens automatically on windows but linux and Pop! does not detect the switch which allows it to know that the laptop os being flipped. Some laptops show up this key , some dont.
ray@pop-os:~/TabletButton$ xinput list
⎡ Virtual core pointer id=2 [master pointer (3)]
⎜ ↳ Virtual core XTEST pointer id=4 [slave pointer (2)]
⎜ ↳ Asus Keyboard id=9 [slave pointer (2)]
⎜ ↳ Asus Keyboard id=11 [slave pointer (2)]
⎜ ↳ ELAN9008:00 04F3:2FC2 id=12 [slave pointer (2)]
⎜ ↳ ASUE120D:00 04F3:31FB Mouse id=14 [slave pointer (2)]
⎜ ↳ ASUE120D:00 04F3:31FB Touchpad id=15 [slave pointer (2)]
⎜ ↳ input-remapper Asus Keyboard forwarded id=20 [slave pointer (2)]
⎜ ↳ ERGO M575 Mouse id=22 [slave pointer (2)]
⎜ ↳ input-remapper ERGO M575 Mouse forwarded id=23 [slave pointer (2)]
⎣ Virtual core keyboard id=3 [master keyboard (2)]
↳ Virtual core XTEST keyboard id=5 [slave keyboard (3)]
↳ Video Bus id=6 [slave keyboard (3)]
↳ Power Button id=7 [slave keyboard (3)]
↳ Sleep Button id=8 [slave keyboard (3)]
↳ Asus Keyboard id=10 [slave keyboard (3)]
↳ ELAN9008:00 04F3:2FC2 Stylus id=13 [slave keyboard (3)]
↳ Asus WMI hotkeys id=16 [slave keyboard (3)]
↳ Asus Keyboard id=17 [slave keyboard (3)]
↳ input-remapper keyboard id=18 [slave keyboard (3)]
↳ input-remapper Asus Keyboard forwarded id=19 [slave keyboard (3)]
↳ input-remapper Asus Keyboard forwarded id=21 [slave keyboard (3)]
We can find the correct input type by disconnecting and connecting. If you are successful and the keyboard is disabled you need an external usb keyboard to start typing to reattach. So its best to use the below script to experiment which finishes in a certain time and you regain keyboard.
#!/bin/bash
#to disconnect by id
#disconnect special key
xinput float 18
#disconnect main keyboard
xinput float 19
#disconnect special key
xinput float 21
#disconnect touch pad
xinput float 15
sleep 10s # Waits 10 seconds.
#Check if your keyboard and touchpad is disabled
#to reconnecct by id to parent
xinput reattach 18 3
xinput reattach 19 3
xinput reattach 21 3
xinput reattach 15 2
Create a Gnome Panel applet to do the same. We need a dependency for this
sudo apt-get install gir1.2-appindicator3-0.1
Now the script
#!/usr/bin/python3
# This code is an example for a tutorial on Ubuntu Unity/Gnome AppIndicators:
# http://candidtim.github.io/appindicator/2014/09/13/ubuntu-appindicator-step-by-step.html
# https://gist.github.com/candidtim/7290a1ad6e465d680b68
import os
import signal
import json
import subprocess
import gi
gi.require_version('Gtk', '3.0')
from gi.repository import Gtk as gtk
from gi.repository import AppIndicator3 as appindicator
from gi.repository import Notify as notify
APPINDICATOR_ID = 'tabletmodeindicator'
def main():
indicator = appindicator.Indicator.new(APPINDICATOR_ID, os.path.abspath('/home/surajitray/TabletButton/tablet.svg'), appindicator.IndicatorCategory.SYSTEM_SERVICES)
indicator.set_status(appindicator.IndicatorStatus.ACTIVE)
indicator.set_menu(build_menu())
notify.init(APPINDICATOR_ID)
gtk.main()
def build_menu():
menu = gtk.Menu()
item_tbmode = gtk.MenuItem('TabletMode')
item_tbmode.connect('activate', tbmode)
menu.append(item_tbmode)
item_lpmode = gtk.MenuItem('LaptopMode')
item_lpmode.connect('activate', lpmode)
menu.append(item_lpmode)
item_quit = gtk.MenuItem('Quit')
item_quit.connect('activate', quit)
menu.append(item_quit)
menu.show_all()
return menu
def tbmode(_):
subprocess.call("/home/surajitray/TabletButton/keyboardtouchpaddisable.sh", shell=True)
return tbmode
def lpmode(_):
subprocess.call("/home/surajitray/TabletButton/keyboardtouchpadenable.sh", shell=True)
return lpmode
def quit1(_):
notify.uninit()
gtk.main_quit()
if __name__ == "__main__":
signal.signal(signal.SIGINT, signal.SIG_DFL)
main()
The scripts are below
keyboardtouchpaddisable.sh
#!/bin/bash
xinput float 18
xinput float 19
xinput float 21
xinput float 15
keyboardtouchpaddisable.sh
#!/bin/bash
xinput reattach 18 3
xinput reattach 19 3
xinput reattach 21 3
xinput reattach 15 2
You can use any svg for the image : /home/surajitray/TabletButton/tablet.svg, just make sure the image and path are correct. Now you can put this item in the startup applications
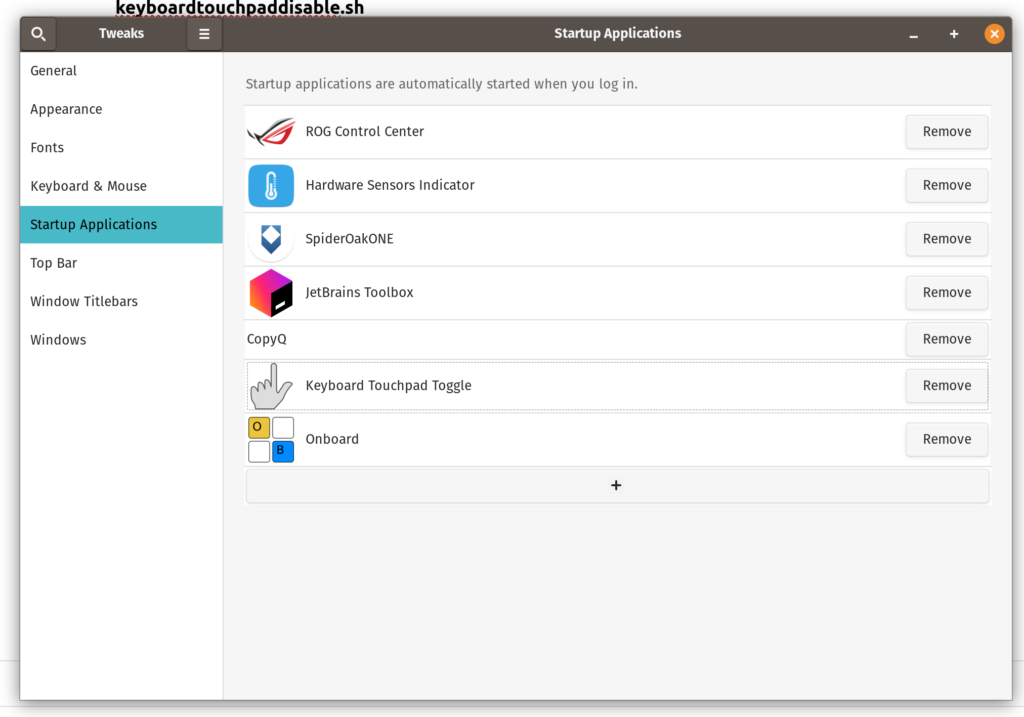